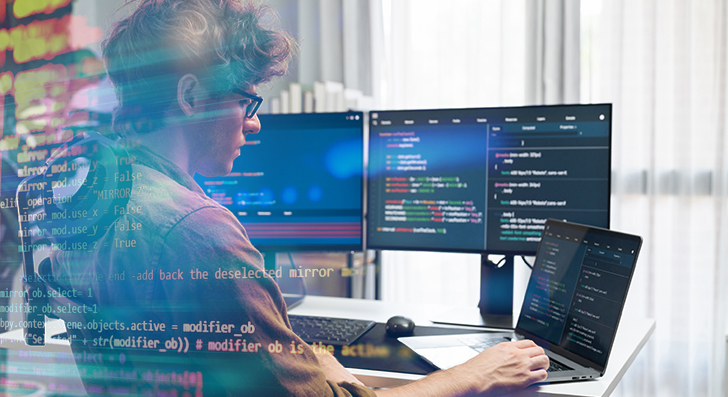
Scalability means your application can deal with growth—extra end users, additional knowledge, and even more visitors—with out breaking. To be a developer, constructing with scalability in mind will save time and anxiety afterwards. Listed here’s a clear and realistic guidebook to assist you start by Gustavo Woltmann.
Layout for Scalability from the Start
Scalability just isn't one thing you bolt on afterwards—it should be aspect of one's approach from the beginning. Lots of programs are unsuccessful after they mature rapidly because the initial design can’t take care of the additional load. As being a developer, you'll want to Believe early regarding how your program will behave stressed.
Commence by designing your architecture to get flexible. Keep away from monolithic codebases where by every little thing is tightly related. Rather, use modular layout or microservices. These styles break your app into more compact, unbiased parts. Each and every module or assistance can scale on its own with out impacting The full procedure.
Also, consider your database from day just one. Will it need to handle 1,000,000 end users or simply just 100? Choose the correct sort—relational or NoSQL—depending on how your facts will grow. Program for sharding, indexing, and backups early, Even when you don’t need them however.
Yet another significant issue is in order to avoid hardcoding assumptions. Don’t publish code that only will work less than present-day conditions. Think about what would occur Should your user base doubled tomorrow. Would your app crash? Would the database slow down?
Use design and style designs that help scaling, like concept queues or occasion-driven systems. These help your application deal with much more requests with out obtaining overloaded.
Whenever you build with scalability in your mind, you are not just planning for achievement—you're lowering potential headaches. A well-prepared procedure is simpler to keep up, adapt, and develop. It’s better to arrange early than to rebuild later on.
Use the appropriate Database
Choosing the right databases is actually a important part of making scalable programs. Not all databases are constructed the identical, and using the Completely wrong one can slow you down or maybe lead to failures as your app grows.
Start out by knowing your information. Can it be remarkably structured, like rows in the table? If Of course, a relational database like PostgreSQL or MySQL is a superb suit. They are potent with associations, transactions, and regularity. Additionally they help scaling procedures like read through replicas, indexing, and partitioning to handle additional site visitors and details.
Should your details is much more adaptable—like user activity logs, merchandise catalogs, or documents—take into account a NoSQL option like MongoDB, Cassandra, or DynamoDB. NoSQL databases are greater at managing big volumes of unstructured or semi-structured facts and can scale horizontally far more easily.
Also, take into consideration your study and produce styles. Have you been executing plenty of reads with less writes? Use caching and browse replicas. Are you handling a weighty generate load? Investigate databases which can deal with substantial produce throughput, or even occasion-based mostly facts storage units like Apache Kafka (for short-term info streams).
It’s also good to Believe forward. You might not will need advanced scaling attributes now, but selecting a database that supports them implies you gained’t will need to modify later.
Use indexing to speed up queries. Steer clear of needless joins. Normalize or denormalize your knowledge dependant upon your entry designs. And generally watch databases effectiveness when you grow.
In short, the proper database depends upon your app’s structure, velocity requires, And exactly how you be expecting it to improve. Acquire time to pick sensibly—it’ll help you save many issues later on.
Optimize Code and Queries
Quick code is key to scalability. As your application grows, just about every smaller delay provides up. Badly created code or unoptimized queries can slow down performance and overload your system. That’s why it’s important to Establish economical logic from the beginning.
Commence by creating clean, very simple code. Avoid repeating logic and take away everything needless. Don’t choose the most advanced Remedy if a simple a person will work. Keep your capabilities short, concentrated, and simple to test. Use profiling instruments to discover bottlenecks—places wherever your code will take too very long to run or takes advantage of excessive memory.
Subsequent, take a look at your databases queries. These frequently gradual issues down much more than the code by itself. Be certain Each and every question only asks for the data you truly require. Prevent Choose *, which fetches everything, and alternatively choose precise fields. Use indexes to speed up lookups. And keep away from accomplishing too many joins, In particular throughout huge tables.
When you notice precisely the same info staying asked for repeatedly, use caching. Keep the effects temporarily employing applications like Redis or Memcached so that you don’t really need to repeat high priced functions.
Also, batch your database operations after you can. Rather than updating a row one by one, update them in groups. This cuts down on overhead and would make your application more effective.
Remember to examination with substantial datasets. Code and queries that do the job fine with 100 information could possibly crash when they have to handle 1 million.
In a nutshell, scalable apps are rapidly applications. Maintain your code restricted, your queries lean, and use caching when wanted. These techniques assistance your software continue to be smooth and responsive, even as the load raises.
Leverage Load Balancing and Caching
As your app grows, it's to deal with a lot more end users and a lot more website traffic. If anything goes by just one server, it will eventually immediately turn into a bottleneck. That’s where load balancing and caching are available. Both of these instruments enable maintain your app quick, stable, and scalable.
Load balancing spreads incoming traffic throughout many servers. As an alternative to one particular server carrying out each of the operate, the load balancer routes end users to distinct servers according to availability. This means no one server will get overloaded. If 1 server goes down, the load balancer can send visitors to the Other folks. Resources like Nginx, HAProxy, or cloud-based methods from AWS and Google Cloud make this very easy to setup.
Caching is about storing info temporarily so it could be reused swiftly. When users ask for the identical info all over again—like a product page or simply a profile—you don’t ought to fetch it in the databases each and every time. You can provide it through the cache.
There are two popular forms of caching:
one. Server-aspect caching (like Redis or Memcached) stores knowledge in memory for quick obtain.
2. Customer-side caching (like browser caching or CDN caching) merchants static files near to the person.
Caching cuts down database load, enhances speed, and can make your application a lot more economical.
Use caching for things that don’t transform frequently. And generally make sure your cache is up-to-date when details does modify.
Briefly, load balancing and caching are basic but powerful equipment. Alongside one another, they help your app take care of more consumers, keep fast, and Recuperate from challenges. If you propose to develop, you may need both of those.
Use Cloud and Container Resources
To make scalable applications, you will need equipment that permit your application grow very easily. That’s the place cloud platforms and containers are available. They offer you flexibility, decrease setup time, and make scaling Considerably smoother.
Cloud platforms like Amazon World-wide-web Products and services (AWS), Google Cloud Platform (GCP), and Microsoft Azure let you lease servers and expert services as you need them. You don’t need to acquire hardware or guess foreseeable future ability. When website traffic improves, you could add more resources with just a few clicks or automatically utilizing auto-scaling. When visitors drops, it is possible to scale down to save cash.
These platforms also offer you companies like managed databases, storage, load balancing, and safety equipment. You'll be able to give attention to developing your app instead of managing infrastructure.
Containers are A further vital Resource. A container deals your app and everything it really should operate—code, libraries, options—into just one unit. This makes it easy to maneuver your app in between environments, from your notebook on the cloud, without having surprises. Docker is the most popular Software for this.
Whenever your app takes advantage of many containers, resources like Kubernetes assist you to regulate them. Kubernetes click here handles deployment, scaling, and Restoration. If one particular element of your application crashes, it restarts it immediately.
Containers also enable it to be very easy to separate portions of your app into products and services. It is possible to update or scale components independently, which happens to be great for general performance and dependability.
In short, employing cloud and container tools suggests it is possible to scale quick, deploy conveniently, and Recuperate immediately when difficulties materialize. If you need your application to develop devoid of limits, start off using these equipment early. They help you save time, minimize possibility, and assist you to keep centered on developing, not repairing.
Keep track of Almost everything
If you don’t check your software, you received’t know when things go Improper. Checking allows you see how your app is doing, location issues early, and make far better selections as your application grows. It’s a vital A part of constructing scalable devices.
Get started by tracking standard metrics like CPU use, memory, disk House, and reaction time. These tell you how your servers and solutions are undertaking. Instruments like Prometheus, Grafana, Datadog, or New Relic will let you obtain and visualize this data.
Don’t just keep track of your servers—check your app way too. Control just how long it will require for people to load internet pages, how frequently glitches materialize, and where by they manifest. Logging tools like ELK Stack (Elasticsearch, Logstash, Kibana) or Loggly will help you see what’s happening inside your code.
Setup alerts for essential issues. For instance, In case your response time goes above a Restrict or simply a company goes down, you'll want to get notified promptly. This can help you correct troubles quickly, usually prior to users even see.
Checking can be beneficial any time you make variations. When you deploy a different attribute and see a spike in faults or slowdowns, you may roll it back again prior to it results in authentic injury.
As your app grows, website traffic and knowledge improve. Without the need of checking, you’ll miss indications of problems until it’s far too late. But with the correct tools in position, you stay on top of things.
In a nutshell, monitoring can help you keep your application dependable and scalable. It’s not pretty much spotting failures—it’s about being familiar with your program and ensuring that it works perfectly, even under pressure.
Remaining Ideas
Scalability isn’t only for large corporations. Even little applications require a robust Basis. By developing carefully, optimizing properly, and utilizing the correct instruments, you are able to build apps that mature easily devoid of breaking under pressure. Commence smaller, Believe massive, and Establish intelligent.